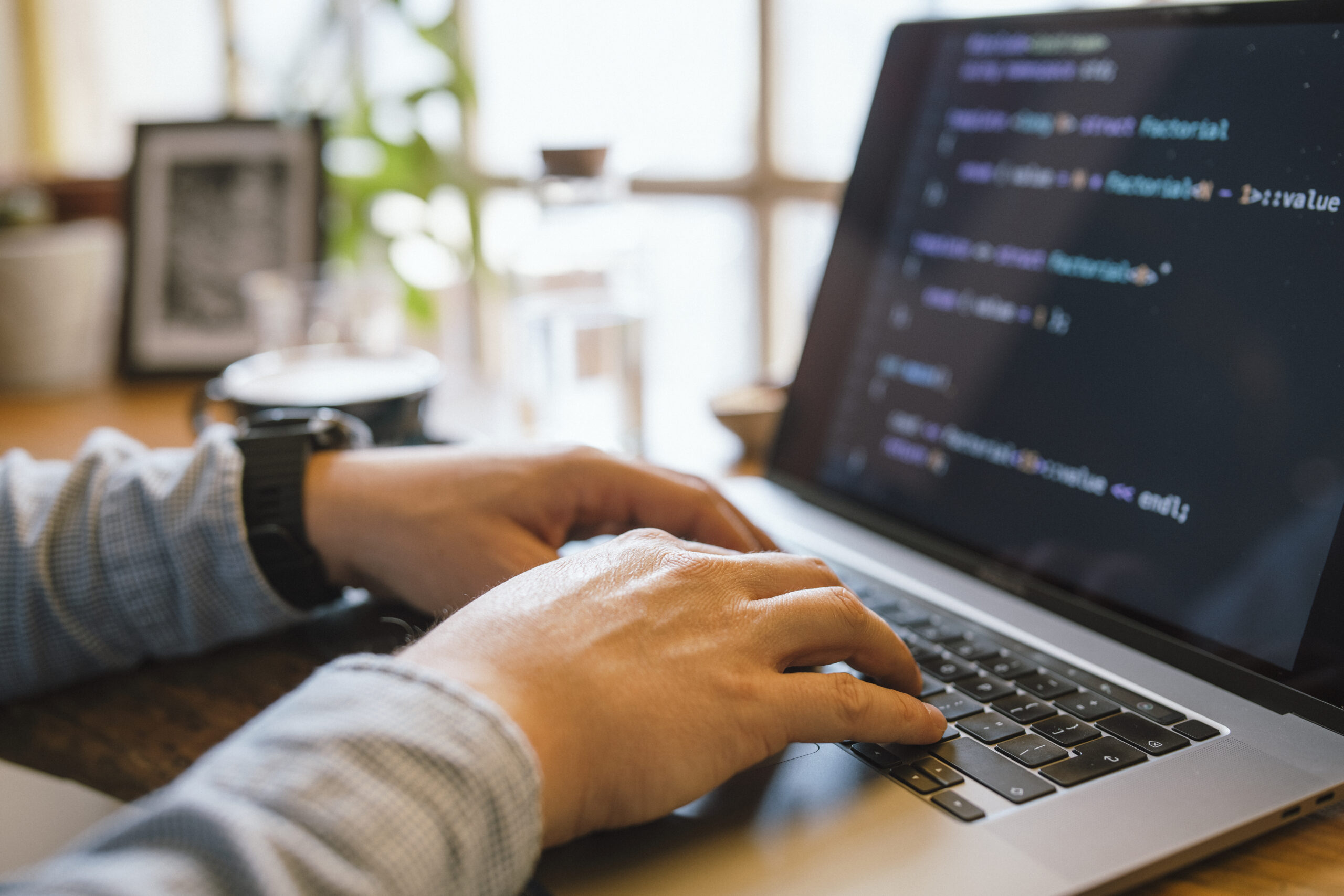
Debugging is Just about the most essential — nevertheless generally missed — abilities within a developer’s toolkit. It is not almost repairing damaged code; it’s about being familiar with how and why things go Incorrect, and Understanding to Consider methodically to resolve troubles competently. Whether or not you're a beginner or a seasoned developer, sharpening your debugging skills can save several hours of frustration and dramatically improve your efficiency. Here i will discuss quite a few procedures that can help developers amount up their debugging activity by me, Gustavo Woltmann.
Learn Your Instruments
Among the list of fastest approaches developers can elevate their debugging abilities is by mastering the tools they use everyday. While crafting code is a person Component of growth, realizing how to connect with it properly in the course of execution is equally vital. Modern-day advancement environments come Geared up with effective debugging capabilities — but quite a few developers only scratch the area of what these equipment can do.
Consider, for example, an Built-in Improvement Ecosystem (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These resources permit you to established breakpoints, inspect the value of variables at runtime, action by means of code line by line, as well as modify code over the fly. When made use of accurately, they let you observe exactly how your code behaves for the duration of execution, that is priceless for tracking down elusive bugs.
Browser developer applications, including Chrome DevTools, are indispensable for entrance-finish builders. They allow you to inspect the DOM, observe network requests, watch genuine-time effectiveness metrics, and debug JavaScript within the browser. Mastering the console, sources, and network tabs can transform irritating UI difficulties into workable duties.
For backend or technique-amount builders, applications like GDB (GNU Debugger), Valgrind, or LLDB provide deep Management around operating processes and memory administration. Studying these instruments can have a steeper Studying curve but pays off when debugging functionality challenges, memory leaks, or segmentation faults.
Beyond your IDE or debugger, grow to be snug with version Handle programs like Git to be familiar with code history, uncover the precise moment bugs had been introduced, and isolate problematic adjustments.
In the long run, mastering your instruments usually means heading over and above default options and shortcuts — it’s about establishing an personal familiarity with your progress ecosystem in order that when troubles occur, you’re not dropped in the dead of night. The higher you already know your instruments, the more time you can spend resolving the particular trouble rather than fumbling by means of the process.
Reproduce the issue
Probably the most crucial — and often missed — methods in productive debugging is reproducing the condition. Right before leaping into the code or producing guesses, builders need to create a regular natural environment or scenario exactly where the bug reliably appears. Without having reproducibility, correcting a bug gets to be a sport of likelihood, usually bringing about wasted time and fragile code changes.
The first step in reproducing a problem is accumulating just as much context as you possibly can. Check with concerns like: What actions led to The difficulty? Which setting was it in — advancement, staging, or production? Are there any logs, screenshots, or mistake messages? The more element you may have, the less complicated it turns into to isolate the exact problems less than which the bug takes place.
Once you’ve gathered adequate info, try to recreate the situation in your local environment. This could signify inputting exactly the same details, simulating equivalent person interactions, or mimicking method states. If The difficulty appears intermittently, consider composing automatic exams that replicate the sting instances or condition transitions concerned. These checks not just assistance expose the issue and also stop regressions Down the road.
Occasionally, The problem might be natural environment-particular — it would take place only on particular running devices, browsers, or below distinct configurations. Using resources like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms is often instrumental in replicating such bugs.
Reproducing the trouble isn’t merely a action — it’s a mindset. It demands persistence, observation, plus a methodical approach. But after you can persistently recreate the bug, you might be now halfway to fixing it. By using a reproducible state of affairs, You should use your debugging equipment far more proficiently, exam prospective fixes safely and securely, and converse more clearly with your team or customers. It turns an abstract complaint right into a concrete challenge — and that’s exactly where developers prosper.
Browse and Have an understanding of the Mistake Messages
Mistake messages tend to be the most respected clues a developer has when one thing goes Improper. As opposed to viewing them as irritating interruptions, builders should really discover to treat mistake messages as direct communications from your method. They frequently tell you exactly what happened, where by it took place, and often even why it occurred — if you know how to interpret them.
Get started by looking at the concept meticulously and in comprehensive. A lot of developers, specially when underneath time stress, look at the main line and promptly commence making assumptions. But further inside the error stack or logs may well lie the real root cause. Don’t just duplicate and paste error messages into search engines like google and yahoo — examine and recognize them initial.
Crack the error down into elements. Can it be a syntax error, a runtime exception, or simply a logic error? Will it stage to a selected file and line quantity? What module or perform activated it? These concerns can tutorial your investigation and stage you towards the responsible code.
It’s also handy to know the terminology of the programming language or framework you’re employing. Error messages in languages like Python, JavaScript, or Java usually abide by predictable patterns, and Mastering to recognize these can substantially speed up your debugging approach.
Some errors are obscure or generic, As well as in Those people situations, it’s very important to examine the context through which the error transpired. Test related log entries, input values, and up to date variations within the codebase.
Don’t forget about compiler or linter warnings either. These usually precede much larger issues and provide hints about likely bugs.
In the long run, mistake messages aren't your enemies—they’re your guides. Understanding to interpret them correctly turns chaos into clarity, assisting you pinpoint troubles speedier, cut down debugging time, and become a a lot more successful and self-assured developer.
Use Logging Correctly
Logging is One of the more potent equipment in a very developer’s debugging toolkit. When made use of correctly, it offers actual-time insights into how an application behaves, serving to you comprehend what’s occurring underneath the hood without having to pause execution or move through the code line by line.
A great logging method starts off with recognizing what to log and at what amount. Popular logging concentrations involve DEBUG, Facts, Alert, Mistake, and Deadly. Use DEBUG for specific diagnostic facts throughout improvement, INFO for general events (like thriving get started-ups), Alert for potential problems that don’t split the appliance, ERROR for real issues, and Deadly if the program can’t proceed.
Keep away from flooding your logs with too much or irrelevant information. Far too much logging can obscure important messages and decelerate your program. Center on critical situations, condition modifications, input/output values, and significant determination details in your code.
Structure your log messages Evidently and continuously. Involve context, for instance timestamps, ask for IDs, and function names, so it’s easier to trace difficulties in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even easier to parse and filter logs programmatically.
For the duration of debugging, logs Allow you to track how variables evolve, what disorders are achieved, and what branches of logic are executed—all without having halting the program. They’re Primarily worthwhile in production environments wherever stepping by way of code isn’t possible.
Moreover, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with checking dashboards.
Finally, smart logging is about equilibrium and clarity. Using a very well-thought-out logging technique, you can lessen the time it will take to spot challenges, acquire further visibility into your purposes, and improve the All round maintainability and trustworthiness of your code.
Assume Similar to a Detective
Debugging is not just a specialized undertaking—it's a kind of investigation. To proficiently identify and resolve bugs, builders should strategy the method similar to a detective resolving a mystery. This state of mind will help stop working advanced issues into workable sections and abide by clues logically to uncover the foundation cause.
Commence by collecting evidence. Consider the indicators of the situation: error messages, incorrect output, or efficiency concerns. Similar to a detective surveys a criminal offense scene, accumulate just as much appropriate facts as you could without the need of jumping to conclusions. Use logs, check circumstances, and user stories to piece jointly a transparent photo of what’s taking place.
Up coming, type hypotheses. Check with on your own: What may very well be resulting in this habits? Have any alterations just lately been created for the codebase? Has this problem occurred just before below similar circumstances? The intention will be to slim down prospects and determine potential culprits.
Then, exam your theories systematically. Endeavor to recreate the condition in a very controlled atmosphere. For those who suspect a certain perform or component, isolate it and confirm if the issue persists. Like a detective conducting interviews, ask your code thoughts and Allow the results guide you closer to the truth.
Fork out near notice to smaller specifics. Bugs usually hide from the least envisioned areas—similar to a missing semicolon, an off-by-a person error, or a race issue. Be comprehensive and patient, resisting the urge to patch The problem without thoroughly comprehending it. Non permanent fixes could disguise the real challenge, only for it to resurface afterwards.
Finally, continue to keep notes on Everything you tried using and discovered. Equally as detectives log their investigations, documenting your debugging process can preserve time for future concerns and assistance Some others comprehend your reasoning.
By contemplating similar to a detective, developers can sharpen their analytical capabilities, approach difficulties methodically, and develop into more practical at uncovering hidden troubles in advanced techniques.
Produce Tests
Creating exams is among the simplest ways to enhance your debugging capabilities and Over-all development effectiveness. Assessments not simply assistance capture bugs early but also serve as a safety net that provides you self confidence when building variations in your codebase. A properly-examined software is simpler to debug as it means that you can pinpoint accurately where and when a problem occurs.
Start with device exams, which concentrate on personal features or modules. These modest, isolated assessments can speedily reveal no matter whether a particular piece of logic is Operating as expected. Every time a examination fails, you quickly know the place to seem, drastically lowering time spent debugging. Device checks are In particular valuable for catching regression bugs—difficulties that reappear immediately after Formerly becoming fixed.
Future, combine integration exams and finish-to-end checks into your workflow. These support make certain that numerous elements of your application do the job alongside one another efficiently. They’re specifically useful for catching bugs that manifest in advanced techniques with multiple parts or providers interacting. If something breaks, your tests can inform you which A part of the pipeline failed and underneath what situations.
Crafting exams also forces you to definitely Consider critically about your code. To test a feature adequately, you require to know its inputs, envisioned outputs, and edge circumstances. This volume of comprehension naturally sales opportunities to better code construction and much less bugs.
When debugging a problem, producing a failing test that reproduces the bug is usually a strong starting point. Once the examination fails continuously, you'll be able to deal with fixing the bug and enjoy your test move when The problem is fixed. This technique makes certain that the identical bug doesn’t return Sooner or later.
In short, creating assessments turns debugging from the irritating guessing video game right into a structured and predictable procedure—supporting you capture extra bugs, more rapidly plus more reliably.
Acquire Breaks
When debugging a tricky concern, it’s uncomplicated to be immersed in the issue—watching your display screen for several hours, attempting Remedy immediately after Alternative. But Just about the most underrated debugging instruments is solely stepping absent. Taking breaks assists you reset your head, cut down frustration, and sometimes see The problem from the new point of view.
When you are far too close to the code for way too very long, cognitive exhaustion sets in. You might begin overlooking apparent problems or misreading code you wrote just several hours previously. In this particular condition, your Mind results in being much less effective at difficulty-fixing. A brief wander, a espresso split, as well as switching to a distinct process for 10–15 minutes can refresh your concentrate. Several developers report discovering the root of a problem when they've taken time to disconnect, permitting their subconscious get the job done from the qualifications.
Breaks also assistance protect against burnout, In particular through more time debugging periods. Sitting before a display screen, mentally caught, is not only unproductive and also draining. Stepping away enables you to return with renewed energy in addition to a clearer frame of mind. You could all of a sudden notice a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you right before.
In case you’re stuck, more info a fantastic rule of thumb would be to established a timer—debug actively for 45–sixty minutes, then take a five–10 moment break. Use that point to move all around, stretch, or do a thing unrelated to code. It may experience counterintuitive, Specially under tight deadlines, but it surely really causes more quickly and more practical debugging In the end.
Briefly, taking breaks is just not an indication of weakness—it’s a wise technique. It offers your Mind Area to breathe, improves your viewpoint, and can help you avoid the tunnel vision That always blocks your development. Debugging is actually a psychological puzzle, and relaxation is part of fixing it.
Study From Every Bug
Every single bug you come upon is more than just A brief setback—It is a chance to mature as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or a deep architectural concern, each can instruct you something useful in case you go to the trouble to reflect and evaluate what went Improper.
Start out by asking your self several essential issues as soon as the bug is resolved: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with far better procedures like unit screening, code testimonials, or logging? The solutions typically expose blind spots in the workflow or understanding and assist you to Construct more powerful coding practices transferring ahead.
Documenting bugs can be a fantastic routine. Hold a developer journal or keep a log in which you Observe down bugs you’ve encountered, the way you solved them, and That which you uncovered. After some time, you’ll begin to see patterns—recurring problems or typical errors—that you could proactively stay away from.
In group environments, sharing Everything you've learned from the bug with the peers may be especially impressive. Irrespective of whether it’s by way of a Slack concept, a brief produce-up, or A fast expertise-sharing session, assisting others steer clear of the very same problem boosts workforce effectiveness and cultivates a more powerful Discovering lifestyle.
Much more importantly, viewing bugs as classes shifts your frame of mind from aggravation to curiosity. In lieu of dreading bugs, you’ll start off appreciating them as important aspects of your advancement journey. In any case, some of the finest developers are certainly not the ones who produce ideal code, but individuals that continually learn from their problems.
Eventually, Every single bug you fix adds a completely new layer in your talent set. So following time you squash a bug, have a second to reflect—you’ll appear absent a smarter, a lot more able developer due to it.
Conclusion
Improving upon your debugging expertise can take time, practice, and persistence — though the payoff is huge. It can make you a far more productive, self-assured, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a possibility to become far better at That which you do.